# Understanding setImmediate()
The Node.js setImmediate function interacts with the event loop in a special way.
When you want to execute some piece of code asynchronously, but as soon as possible, one option is to use the setImmediate()
function provided by Node.js:
setImmediate(() => {
//run something
})
Any function passed as the setImmediate() argument is a callback that’s executed in the next iteration of the event loop.
How is setImmediate()
different from setTimeout(() => {}, 0)
(passing a 0ms timeout), and from process.nextTick()
?
A function passed to process.nextTick()
is going to be executed on the current iteration of the event loop, after the current operation ends. This means it will always execute before setTimeout
and setImmediate
.
A setTimeout()
callback with a 0ms delay is very similar to setImmediate()
. The execution order will depend on various factors (opens new window), but they will be both run in the next iteration of the event loop.
setImmediate
callbacks are called after I/O Queue callbacks are finished or timed out. setImmediate callbacks are placed in Check Queue, which are processed after I/O Queue.
setTimeout(fn, 0)
callbacks are placed in Timer Queue and will be called after I/O callbacks as well as Check Queue callbacks. As event loop, process the timer queue first in each iteration, so which one will be executed first depends on which phase event loop is.
# Event Loop
Event loop handles all async callbacks, starting from timers to close callbacks. There is also a nextTickQueue in the middle, however it’s not a part of the event loop itself. Each phase has a queue attached to it. When event loop enters in a particular phase, its target is to execute the callbacks/tasks in those queues.
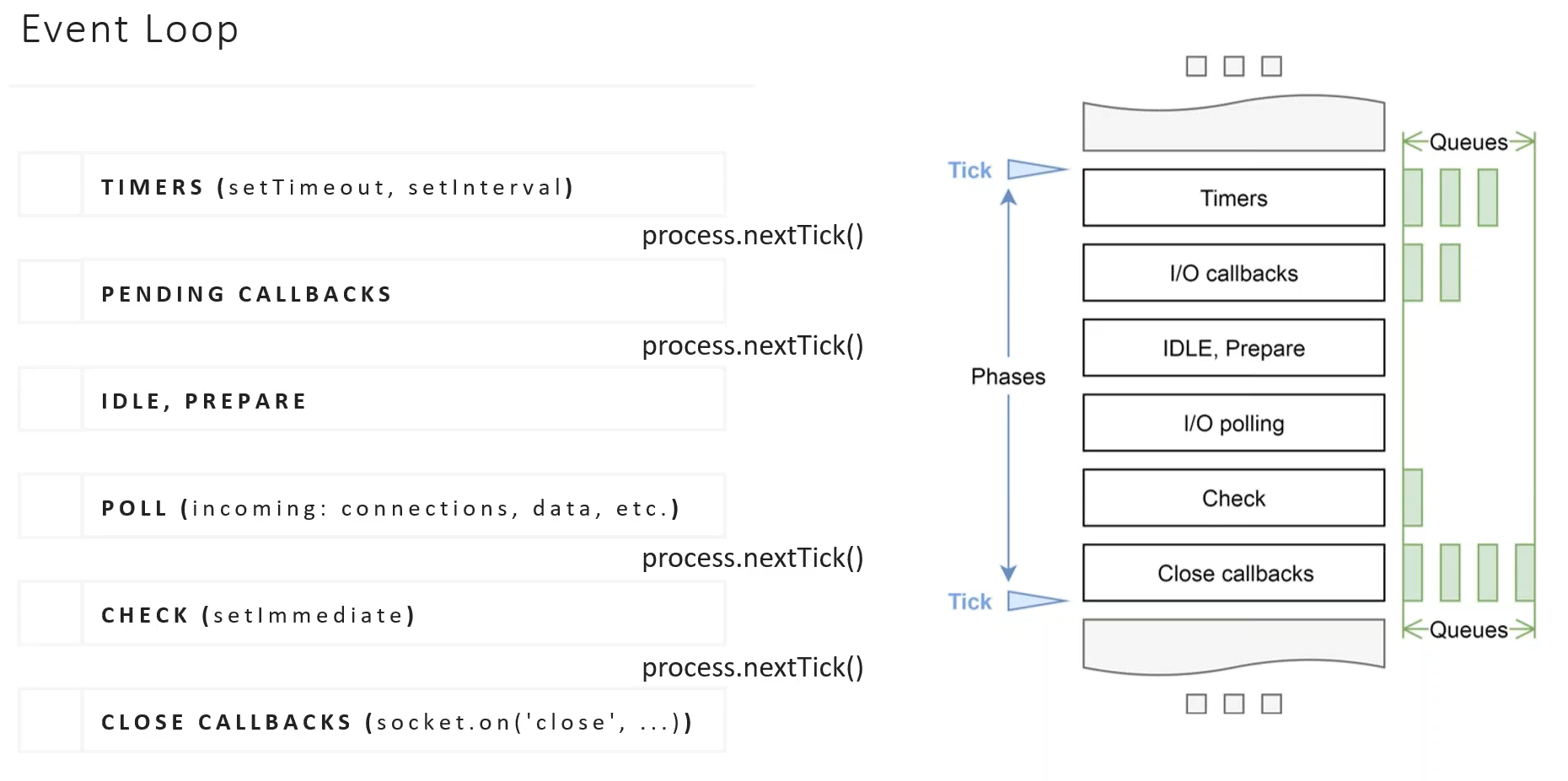
Phase | Description |
---|---|
Timer | It handles the callbacks assigned by setTimeout & setInterval after the given time threshold is completed. |
I/O callbacks | Handles all callbacks except the ones set by setTimeout, setInterval & setImmediate. It also does not have any close callbacks. |
Idle, prepare | Used internally |
Pole | Retrieve new I/O events. This is which makes node a cool dude. |
Check | Here the callbacks of setImmediate() is handled. |
Close callbacks | Handles close connection callbacks etc. (eg: socket connection close) |
nextTickQueue | Holds the callbacks of process.nextTick(); but not a part of the event loop. |
# 参考资料
[1] setImmediate() vs nextTick() vs setTimeout(fn,0) - in depth explanation (opens new window)
