# Clean Code
Why does bad code get written? Sometimes you're just trying to complete a task on time. You know it's not your best work, but the program is running and you're relieved. You tell yourself you'll clean it up later, but "later" never comes.
Maintenance doesn't just start after product launch - it happens throughout the development phase. Having clean code is critical to making maintenance as efficient and simple as possible. Developers spend 60% of their time trying to understand code before making any changes.
Clean code complies with the DRY rule (Don’t Repeat Yourself). From what I understand, these clean code approaches implement the DRY rule. Again, this ease comes from following popular programming principles like KISS (Keep It Simple, Stupid) and YAGNI (You Ain't Gonna Need It).
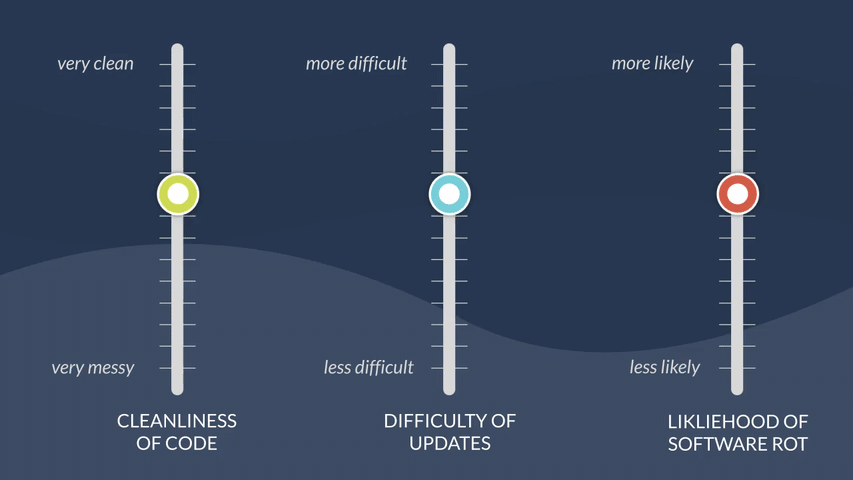
# Naming
Rules of Naming |
---|
The name of a variable, function, or class should tell you three things: why it exists, what it does, and how it is used. |
One Word, One Meaning |
Avoid number prefixes |
Use pronounceable and meaningful names |
Rules of Length |
---|
The longer the scope of a function, the shorter its name should be.(low-level code) |
The shorter the scope of a function, the longer its name should be. |
Local variables of a small block should have short names. |
Grammar Rules |
---|
Class and Object Names: Use noun phrase names |
Method Names: Use verb phrase names |
Method and Class Length: Long scope = Short name, Short Method scope = Long name |
# Functions
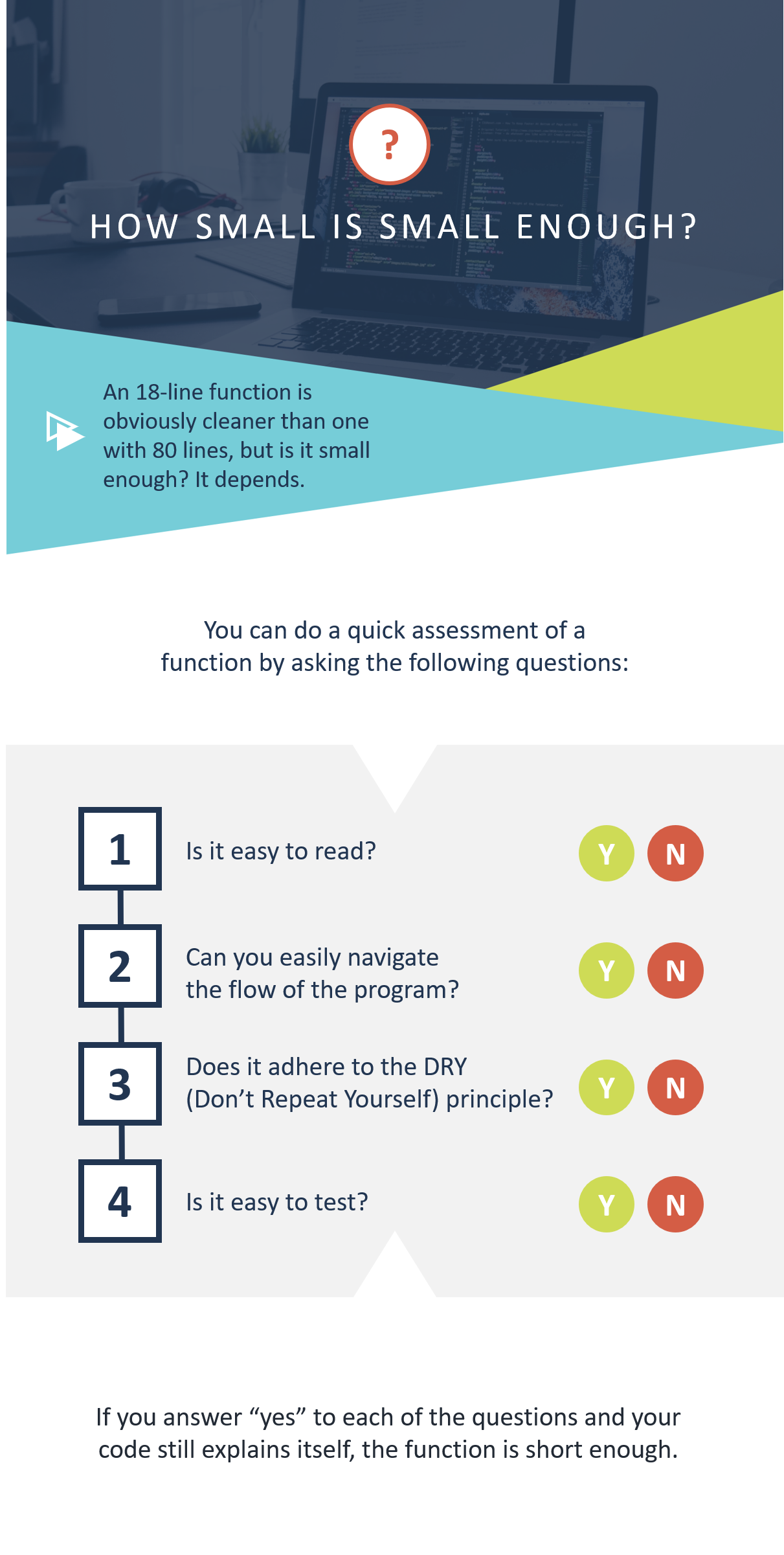
Good Function? |
---|
Functions should only do one thing. |
Functions should be a command or a query - Not both. |
Functions should have meaningful names. |
Functions should not have side effects. |
# The "Golden Number" of Function Parameters
The “golden number” is between zero and two parameters. There are rare cases when you may need three, but that should not be the norm. Regardless of whether your function has zero or three parameters, it is your responsibility to make sure the code can be read quickly and easily by other developers.
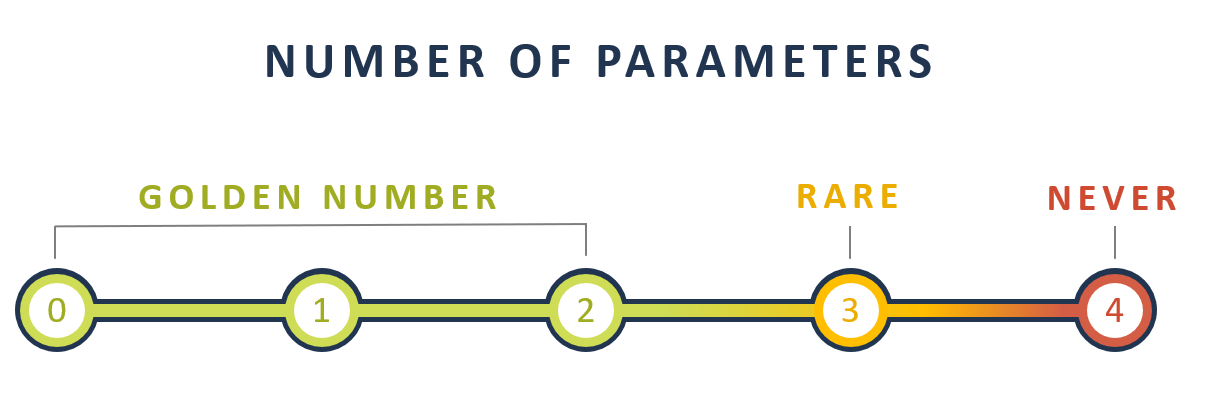
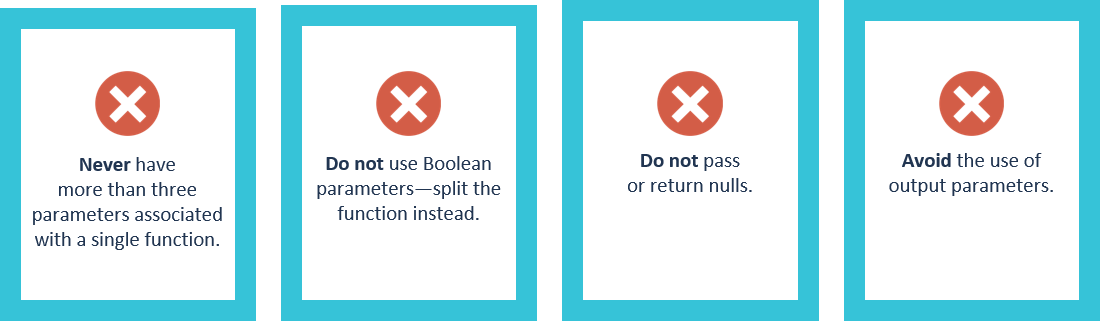
# Side Effects
It is a best practice to minimize the use of side effects as much as possible. When you have no choice but to use a side effect, you can utilize the block passing technique to minimize the disruption and maintain the readability of your code.
Pros | Cons |
---|---|
Side effects allow code to make changes to DB or write to external files | Side effects can make a function return a different return each time it's called |
To work around the paired coupling of side effects, use the “passing the block” technique; this eliminates the issues caused by temporal coupling. | Side effects can obscure the logic, decrease the readability |
# Comments
You may have heard the saying, “Good code is self-documenting.” This is true of all code, but it is especially true of clean code.
One way to ensure code is self-documenting is by using comments. Comments are brief summaries that explain code in simple text and are often referred to by developers when code is refactored.
The Good
- Legal Comments
- Informative Comments
- Explanation of Intent
- TODO Comments
- Improving the Code
The Bad
- Irrelevant Comments
- Bad Code
- Redundant Comments
- Commented Out Code
- Journaling Comments
- Noise Comments
- Closing Brace Comments
# DRY vs WET
DRY—Don’t Repeat Yourself—is a software coding principle focused on reducing the duplication of information. Using DRY supports maintenance efforts, readability of code, and saves time and money.
WET—Write Every Time—is a term used to describe when the dry principle is not applied to code. Essentially, WET code causes the need to write code repeatedly due to maintenance and reusability challenges caused by code that doesn’t follow the DRY principle.
Sometimes it doesn’t make sense to keep our code DRY. Here are some examples when it makes sense to violate the DRY principle.
- Performance
- By introducing duplication, we can improve performance. The key here is to control the scope of duplication, like introducing it only to one place where a performance boost is needed.
- Deduce Coupling
- We introduce some duplication to reduce component coupling. For example, we can have a dedicated data access layer for every microservice instead of creating one shared data access layer which makes all microservices couple to it.
- Tests
- The readability of a test is more important than possible duplication. Keeping in mind that in most cases duplication is a bad thing, we still can find tests where duplication is warranted in order to express the "test case".
- Automatically Generated Code
- Code that is automatically generated can have a lot of duplications. Even though this is true, it’s important not to manually change the code. Over time, manually changing generated code will make it difficult, if not impossible, to replace the code automatically.
Enforcing DRY Programmers often develop code in teams. While a team dynamic works well for writing large amounts of code, it can make it difficult to maintain code that is free of duplications.
- Reviews
- Regular code reviews are one of the most effective ways to detect already introduced DRY violations.
- Tools
- Modern static code analysis tools support the detection of duplicated pieces of code. like SonarQube
- Documentation
- Knowledge Sharing
- Team Culture
- Set up a proper team culture by educating team members on the importance of avoiding duplications.
# Conclusion
At this point, you should understand what clean code is and why it is important. By the clean code learned, let's write elegant code from now on.
