# JSON.stringify()
The JSON.stringify() method converts a JavaScript object or value to a JSON string.
We all know that console.log()
can not print an object directly. It prints [object object]
. So we use JSON.stringify()
to first convert the object into a string and then print in the console, like this.
Generally, developers use this stringify
function in a simple way as we did above. But I am gonna tell you some hidden secrets of this little gem which will make your life easy.
# 1. The second argument(Array)
Yes, stringify
function can have a 2nd argument also. It's an array of keys to the object which you want to print in the console.
In this way, you can ignore these useless keys, focus on the keys you want to know. Instead of printing the whole JSON object we can print only the required key by passing it as an array in the 2nd argument.
# 2. The second argument(Function)
We can also pass a 2nd argument as a function. It evaluates each key-value pair according to the logic written in the function. If you return undefined
the key-value pair will not print. See this example for a better understanding.
Only id
is printed as our function condition return undefined
for the value typeof
string.
# 3. The third argument as Number
The third argument controls the spacing in the final string. If the argument is a number, the print result will expand and indent the characters of this number.
This feature is very helpful for our local development. According to the digital size, the corresponding indentation length can also be adjusted.
# 4. The third argument as String
If the third argument is a string, it will be used instead of the space character as displayed above.
Here '-' replace the space character. Sometimes you can also use this style to print results.
# 5. The toJSON method
We have one method named toJSON
which can be part of any object as its property. JSON.stringify()
returns the result of this function and stringifies it instead of converting the whole object into the string. See this example.
Here we can see instead of printing the whole object, it only prints the result of toJSON
function.Generally, this scheme can filter out some database fields that are not needed by the front end.
# 6. Colorful Console Message
If you’re running node.js application, you can use the color reference of text to style your messages.
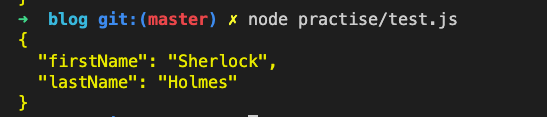
%s
is where in the string (the second argument) gets injected. \x1b[0m
resets the terminal color so it doesn't continue to be the chosen color anymore after this point.
# 7. Summary
In this section we learned about these interesting features of JSON.stringify()
. I hope you can remember and use them in certain required scenarios.
